Angular Mobile App Development leverages the Angular framework to create efficient and interactive mobile applications. It utilizes modern web technologies for a native-like user experience.
Angular, a platform built on TypeScript, offers developers a robust environment for crafting mobile apps that deliver high performance and rich features. By embracing the Angular framework, developers can harness the power of its comprehensive toolset to construct applications that are not only responsive but also maintainable and scalable.
The framework’s ability to work seamlessly with other libraries and its robust ecosystem make it a popular choice for mobile app development. Angular has the advantage of a modular architecture, simplifying the development process and allowing for code reuse. This leads to faster development cycles and a quicker time to market for mobile apps. Angular’s data binding and dependency injection features also help create dynamic and efficient applications that adapt quickly to users’ evolving needs.
Introduction To Angular Mobile App Development For Mobile Apps
Welcome to the world of mobile app development with Angular. This powerful framework offers developers the tools to create dynamic, high-performance applications for mobile devices. With its robust features and ease of use, Angular has become a go-to choice for developers looking to build standout mobile apps.
Why Choose Angular
Angular is a versatile framework ideal for mobile app development. Below are key reasons to choose Angular:
- Consistent code structure makes apps easy to maintain.
- Component-based architecture enhances app performance.
- Two-way data binding ensures seamless data synchronization.
- Angular CLI simplifies project management and scaffolding.
- Mobile-specific features with Ionic Framework integration.
Comparing Angular With Other Frameworks
Selecting the proper framework is crucial for project success. Here’s a comparison:
Framework | Performance | Learning Curve | Community Support |
---|---|---|---|
Angular | High | Moderate | Extensive |
React Native | High | Moderate to Steep | Extensive |
Flutter | High | Steep | Growing |
Angular stands out with its balance of performance and community support, making it a reliable choice for mobile app projects.
Setting The Foundation
Angular mobile app development begins with a solid foundation. You need the right tools and a clear plan like building a house. Let’s prepare for a smooth development journey.
Installing Necessary Tools
Start with the basics:
- Node.js: The runtime environment.
- NPM: The package manager will install libraries.
Install Angular CLI:
npm install -g @angular/cli
Check the installation:
ng version
Understanding Angular’s Structure
Angular uses a component-based architecture.
Component | Role |
---|---|
Modules | Organize code into blocks. |
Components | Define views. |
Services | Handle data and logic. |
Templates | Provide HTML markup. |
Explore the project folder:
- Src/app: Your code lives here.
- Src/assets: Static files like images go here.
- Src/environments: Set up different environments here.
Designing A User-friendly Interface
Designing a User-Friendly Interface is the heart of successful Angular mobile app development. A seamless and intuitive interface ensures user engagement and satisfaction. With its robust framework, Angular provides developers with tools to create such an environment.
Leveraging Angular Material
Angular Material offers a set of reusable UI components. These components help developers build consistent, attractive interfaces quickly. Angular Material follows Material Design principles and offers:
- Pre-built components like buttons, checkboxes, and sliders
- Customizable themes to match your brand
- Accessibility features for all users
You can use Angular Material to ensure your app looks great and functions nicely across different devices and platforms.
Responsive Layouts With Flex-layout
Flex-Layout is a layout API for Angular. It allows for the creation of responsive designs with ease. With Flex-Layout, developers can:
- Arrange elements on a page with flexibility
- Adjust layouts for different screen sizes
- Control the sizing, positioning, and spacing of elements
Responsive layouts are critical in providing a consistent experience across all devices. Flex-Layout simplifies this process, making your Angular app adaptable to any screen.
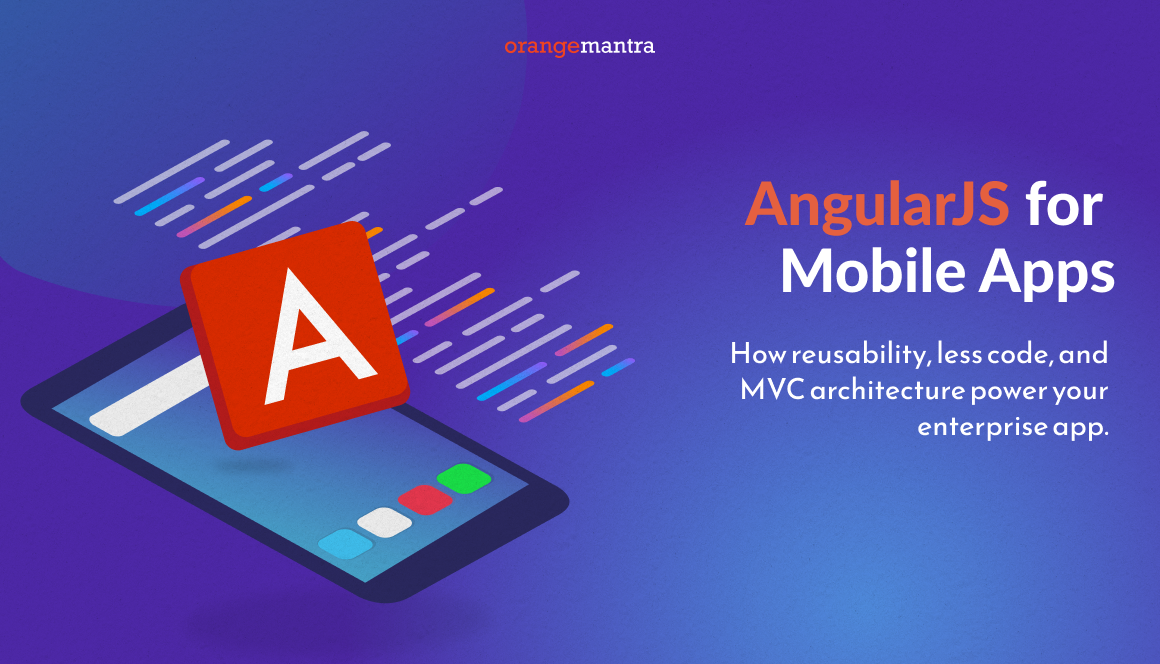
Credit: www.orangemantra.com
Deep Dive Into Angular Components
Welcome to our deep dive into Angular components. This section explores the building blocks of Angular mobile app development.
Creating Components
Angular components form the core of every Angular application. A component controls a patch of the screen called a view. To create a component, you must define a class that includes properties and methods. Here is a simple guide to creating components:
- Set up your development environment.
- Create a new Angular project using Angular CLI.
- Generate a new component using the command:
ng generate component component_name
. - Develop the component class, template, and styles.
Each component includes an HTML template that declares what is rendered on the page. The TypeScript class defines behavior, and styles are represented in CSS.
Managing Component Interaction
In Angular, components often work together. Managing how these components interact is crucial for a robust application. Here are vital methods to manage component interaction:
- Input – allows data to flow from a parent to a child component.
- Output – emits data from a child to a parent component.
- View encapsulation – controls CSS scoping.
For example, if you want a child component to send data back to its parent, you can use the @Output()
decorator and an EventEmitter
.
Method | Use Case |
---|---|
Input | Send data to the child component |
Output | Receive data from child component |
State Management In Angular
Managing an application’s state is crucial for tracking everything in a mobile app. In Angular, state management is a complex but vital piece of the puzzle, ensuring the app runs smoothly and efficiently. Understanding how to handle state can improve your app’s performance and user experience.
Benefits Of Ngrx
NgRx is a powerful library for managing state in Angular applications. It’s built on Redux principles, which help to maintain consistency across your app.
- Predictability: With NgRx, the state transitions are predictable and easy to track.
- Performance: NgRx optimizes performance by using immutable data structures.
- Debugging: The Redux DevTools extension allows developers to inspect state changes over time.
- Maintainability: NgRx’s structured approach to state management simplifies maintaining large applications.
Simplifying State With Akita
Akita is another state management solution that emphasizes simplicity and ease of use. While it may not be as well-known as NgRx, it offers some compelling features for Angular developers.
- Less Boilerplate: Akita requires fewer lines of code, making it easier to implement.
- Flexibility: It provides more straightforward APIs for everyday tasks, offering flexibility in usage.
- Built-in Store: Akita has a store and a query system that simplifies data retrieval and manipulation.
- Entity Management: Handling collections of data is more intuitive with Akita’s entity service.
Optimizing Performance For Mobile
Performance is key when building mobile apps with Angular. Users expect fast, responsive applications on their devices, and optimizing your Angular app ensures a smoother experience. Here are essential strategies to boost your mobile app’s speed and efficiency.
Lazy Loading Modules
Lazy loading is a design pattern that dramatically improves app speed. It loads features only when needed. This means a faster initial load time and reduced resource consumption.
- Break your app into distinct feature modules.
- Configure routes to load modules on demand.
- Use Angular’s built-in support for lazy loading.
With lazy loading, users enjoy a snappy app startup. They won’t wait for unnecessary code to load.
Effective Use Of Caching
Caching is a powerful technique for storing data for future requests. It reduces server calls and data processing.
Type of Caching | Benefits |
---|---|
Service Worker Caching | Enables offline capabilities and faster resource retrieval. |
HTTP Caching | Reduces latency and saves bandwidth. |
Application Data Caching | Speeds up the application by storing frequently used data. |
Implement caching strategies that align with your app’s needs. Your app will become more reliable and performant.
Integrating With Mobile Features
Developing mobile apps with Angular opens many doors, especially when integrating mobile features. Angular’s robust framework helps tap into native mobile capabilities and manage offline functionalities efficiently. Let’s dive into how Angular makes this possible.
Accessing Native Device Capabilities
Angular apps can interact directly with mobile devices. This interaction enhances user experience significantly. Here’s how Angular achieves this:
- Using Cordova/PhoneGap: These frameworks extend Angular apps into mobile apps, accessing device features like camera, GPS, and more.
- Plugins: Numerous plugins are available that unlock features specific to mobile devices.
- NativeScript: This open-source framework allows Angular to use native APIs directly.
By leveraging these tools, developers can build applications that are not only powerful but also intuitive and responsive to user needs.
Handling Offline Functionality
Ensuring app functionality offline is crucial for user retention. Angular provides effective solutions:
- Service Workers: They allow apps to load and perform tasks offline.
- PWA Capabilities: Angular supports Progressive Web Apps, which operate offline and offer an app-like experience.
- Caching Strategies: Implementing advanced caching helps maintain data and UI state.
These features ensure the app remains functional without an internet connection, providing a seamless user experience.
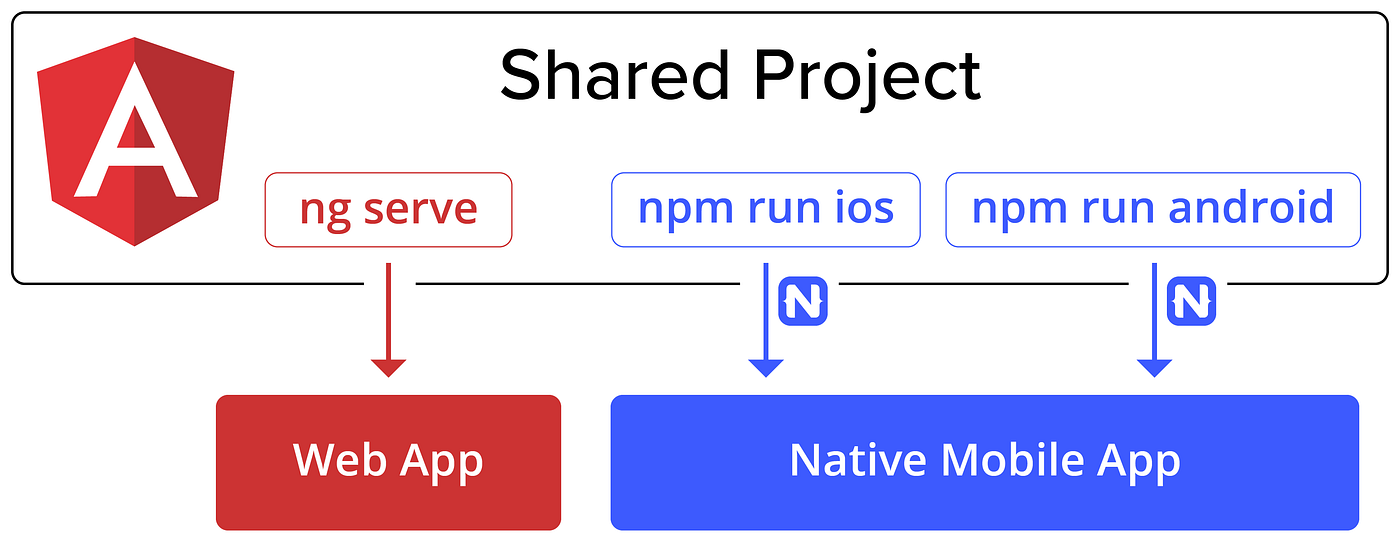
Credit: blog.angular.io
Testing And Debugging
Testing and debugging are crucial for building robust Angular mobile apps. They ensure your application runs smoothly. A well-tested app offers a great user experience. In this section, we dive into two essential testing methods: unit testing and end-to-end testing.
Unit Testing With Jasmine And Karma
Unit testing checks individual parts of your code. Angular pairs well with Jasmine for writing test cases. Karma is the test runner that executes these tests in a real browser.
Critical steps for setting up Jasmine and Karma include:
- Install Jasmine and Karma via npm.
- Configure the Karma test runner.
- Write test cases with Jasmine syntax.
- Run tests to check code functionality.
Here’s a basic test example:
describe('AppComponent', () => {
it('should create the app', () => {
const fixture = TestBed.createComponent(AppComponent);
const app = fixture.debugElement.componentInstance;
expect(app).toBeTruthy();
});
});
This test checks if the AppComponent is created successfully.
End-to-end Testing With Protractor
End-to-end testing simulates user interactions across the entire app. A protractor is a powerful tool designed for Angular apps.
Follow these steps for end-to-end testing:
- Install Protractor using npm.
- Write test scenarios that mimic user actions.
- Run these scenarios against your Angular mobile app.
Example of a Protractor test:
describe('Protractor Demo App', () => {
it('should add one and two', () => {
browser.get('http://juliemr.github.io/protractor-demo/');
element(by.model('first')).sendKeys(1);
element(by.model('second')).sendKeys(2);
element(by.id('gobutton')).click();
expect(element(by.binding('latest')).getText()).toEqual('3');
});
});
This test adds two numbers in a demo app and checks the result.
Deploying Your Angular App
Now that you’ve built your Angular mobile app, it’s time to share it with the world. Deploying your Angular app is the final step in the development process. This guide will help you prepare your app for production and get it into the app stores.
Building For Production
First things first, let’s build your app for production. This step optimizes your app for the best performance. Here’s how:
- Open your terminal.
- Navigate to your project directory.
- Run the build command:
ng build --prod
.
The –prod flag is crucial. It turns on the production mode, which enables various optimizations. These include minification and tree shaking. Minification reduces file size, and tree shaking removes unused code. Both improve your app’s speed.
After the build, you will find the output in the /dist
folder. This folder contains all the files you need to deploy.
Publishing To App Stores
Ready to see your app on user devices? Follow these steps:
Step | Action | Details |
---|---|---|
1 | App Store Accounts | Create developer accounts on platforms like Google Play and the Apple App Store. |
2 | App Preparation | Assemble all the necessary metadata, such as your app’s title, description, and keywords. |
3 | App Submission | Upload your app’s .apk or .ipa file and metadata to the stores. |
4 | Review Process | Wait for the app store’s approval. This can take several days. |
5 | Launch | Once approved, your app goes live. Users can now download and install it. |
Remember: Each store has its guidelines. Always check these before submission. This ensures a smooth approval process.
- Google Play often requires less time to approve apps than the Apple App Store.
- Keep your app’s content and functionality aligned with the platform’s policies.
- Respond promptly to any issues raised during the review process.
Deploying your Angular app might seem daunting. But with this guide, it’s just a few steps away. Get ready to showcase your app to the world.
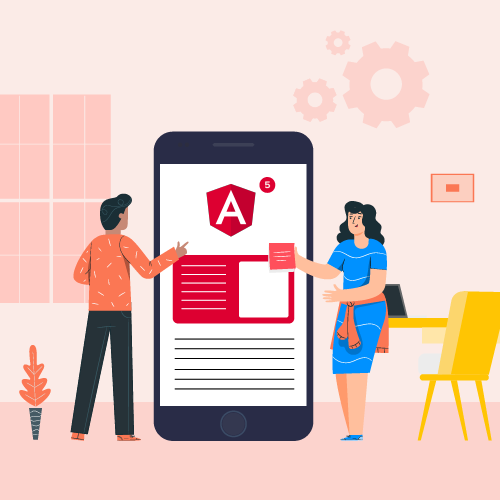
Credit: appinventiv.com
Maintaining And Updating Your App
After launching an Angular mobile app, the journey does not end. Regular maintenance and updates are crucial. They keep the app competitive and secure, ensuring users enjoy the best experience. Let’s explore the essential steps for maintaining and updating your Angular mobile app.
Implementing Continuous Integration/continuous Deployment
Continuous Integration (CI) and Continuous Deployment (CD) are essential to modern app development. CI/CD automates the process from code change to production. This saves time and reduces human error. Below are steps to implement CI/CD:
- Set up a source control repository. This tracks all code changes.
- Automate tests. Ensure the new code does not break the app.
- Configure build servers. They compile and build the app automatically.
- Deploy automatically to staging. Test in a production-like environment.
- Release to production. After successful tests, update the live app.
Monitoring App Performance And User Feedback
Keeping an eye on how your app performs is crucial. User feedback is a gold mine for improvements. Here’s how to stay on top of both:
Performance Monitoring | User Feedback |
---|---|
|
|
You keep your Angular mobile app at its best by monitoring and acting on performance data and user feedback. This leads to happy users and a successful app.
Frequently Asked Questions
Can I Use Angular For Mobile App Development?
You can use Angular for mobile app development by leveraging frameworks like Ionic or NativeScript, which integrate with Angular to build cross-platform mobile applications.
Can I Build An App With Angular?
Yes, you can build an app using Angular, a robust platform for developing efficient and sophisticated single-page applications.
Is Angular Mobile Friendly?
Yes, Angular is mobile-friendly. It supports responsive design and is compatible with various mobile devices, enhancing user experience on smartphones and tablets.
Is Flutter Similar To Angular?
No, Flutter and Angular are not similar. Flutter is a UI toolkit for building natively compiled mobile, web, and desktop applications from a single codebase. Angular, on the other hand, is a platform for building mobile and desktop web applications.
They serve different development purposes.
Conclusion
Embracing Angular for mobile app development offers clear advantages. It streamlines processes, enhances user experience, and integrates seamlessly with existing systems. As technology evolves, Angular remains reliable and powerful for building robust mobile applications. Consider adopting Angular to elevate your app development strategy today.

Driven by a passion for innovation, I am a seasoned tech specialist dedicated to pushing the boundaries of possibility. With 5 years of experience in the ever-evolving landscape of technology, I have honed my expertise in Software Development, Artificial Intelligence, Machine Learning, Drone Technology, 3D Modeling, Automation, Smart Gadgets etc . I am thrived in dynamic environments where creativity meets technical proficiency. My commitment to staying at the forefront of technological advancements ensures that I am not only adapt to change but lead it, shaping the future of tech one breakthrough at a time.